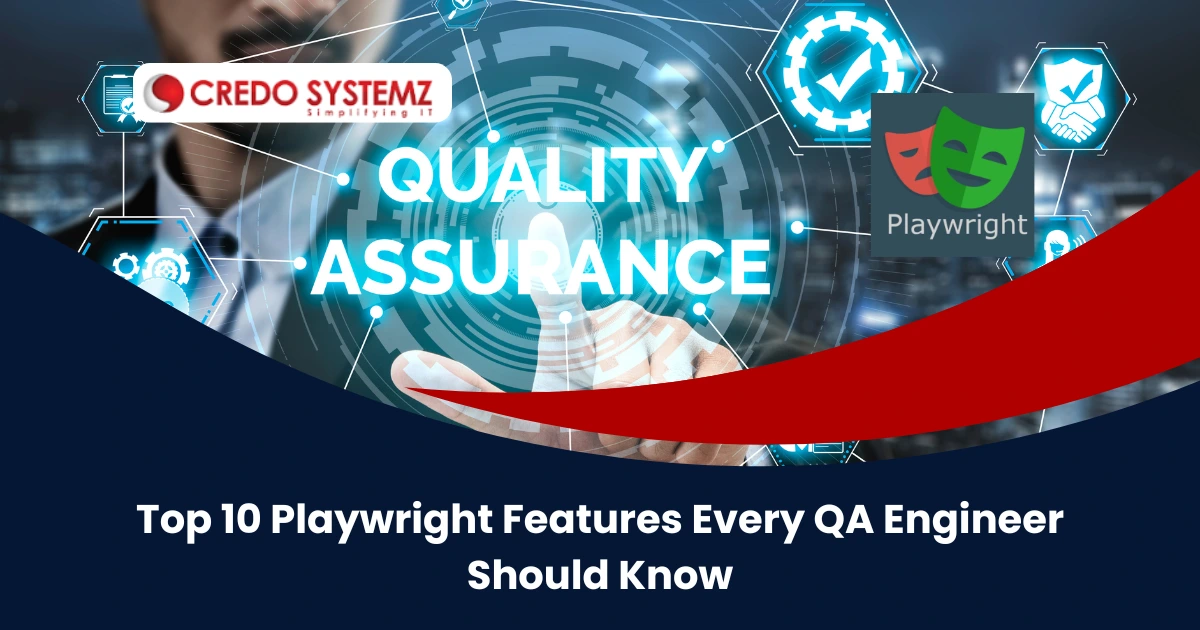
Introduction
In the tech industry, Playwright is one of the popular open-source automation frameworks which offers an all-in-one solution for web automation testing. It stands out from the rest with its speed, reliability, and cross-browser capabilities. Mastering Playwright’s key features can significantly enhance your testing career.
Cross-Browser and Cross-Platform Testing
Playwright supports different browsers like Chromium, WebKit, and Firefox to run tests effortlessly. It offers cross-platform compatibility and works on Windows, macOS, and Linux.
Example: Running tests on different browsers:
const { chromium, firefox, webkit } = require('playwright'); (async () => { for (const browserType of [chromium, firefox, webkit]) { const browser = await browserType.launch(); const context = await browser.newContext(); const page = await context.newPage(); await page.goto('https://example.com'); console.log(await page.title()); await browser.close(); } })();
Auto-Waiting for Elements
Playwright can automatically wait for elements to be ready before interacting with them. This eliminates the need for explicit waits.
Example:
await page.click('button#submit'); // Waits until the button is clickable
Headless and Headed Mode Execution
In a headless mode, Playwright runs tests by default and also supports headed mode for debugging.
Example: Running tests in headed mode:
Powerful Network Interception
To intercept network requests and responses, Playwright can be used. It also makes it easy to mock API calls and test different scenarios.
Example: Mocking a network request:
await page.route('**/api/users', route => { route.fulfill({ status: 200, contentType: 'application/json', body: JSON.stringify({ users: [{ id: 1, name: 'John Doe' }] }), }); });
Robust Debugging Capabilities
Playwright provides rich debugging tools to help analyze and debug test runs like, Playwright Inspector and trace viewer.
Example: Enabling debugging mode:
Multiple Tabs and Contexts Handling
Playwright is efficient in handling multi-user scenarios by allowing testing multiple browser contexts or tabs simultaneously.
Example: Opening multiple tabs:
const context = await browser.newContext(); const page1 = await context.newPage(); const page2 = await context.newPage(); await page1.goto('https://example.com'); await page2.goto('https://playwright.dev');
Screenshot and Video Recording
Playwright helps with debugging and documentation by supporting the process of taking screenshots and recording videos.
Example: Taking a screenshot:
await page.screenshot({ path: 'screenshot.png' });
Example: Recording a video:
const context = await browser.newContext({ recordVideo: { dir: 'videos/' } });
Built-in Test Runner with Parallel Execution
Playwright supports parallel execution with built-in test runners and reduces the test execution time.
Example: Running tests in parallel
npx playwright test --workers=4
API Testing Capabilities
Along with UI testing, Playwright also supports API testing to eliminate the need for separate tools like Postman.
Example: API request and response validation:
const response = await page.request.get('https://api.example.com/data'); console.log(await response.json());
Support for Mobile Emulation
Playwright supports mobile emulation by testing web apps on the mobile devices by simulating different screen sizes and user agents.
Example: Emulating a mobile device:
const context = await browser.newContext({ viewport: { width: 375, height: 812 } });
Conclusion
To sum up, Playwright is the best solution for modern test automation that offers robust features for cross-browser testing, network interception, debugging, parallel execution, and more. To test web applications, APIs, or mobile web views, learn the Playwright skills by joining our playwright training course. Master the top features of playwright and enhance your QA testing career journey.
Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.