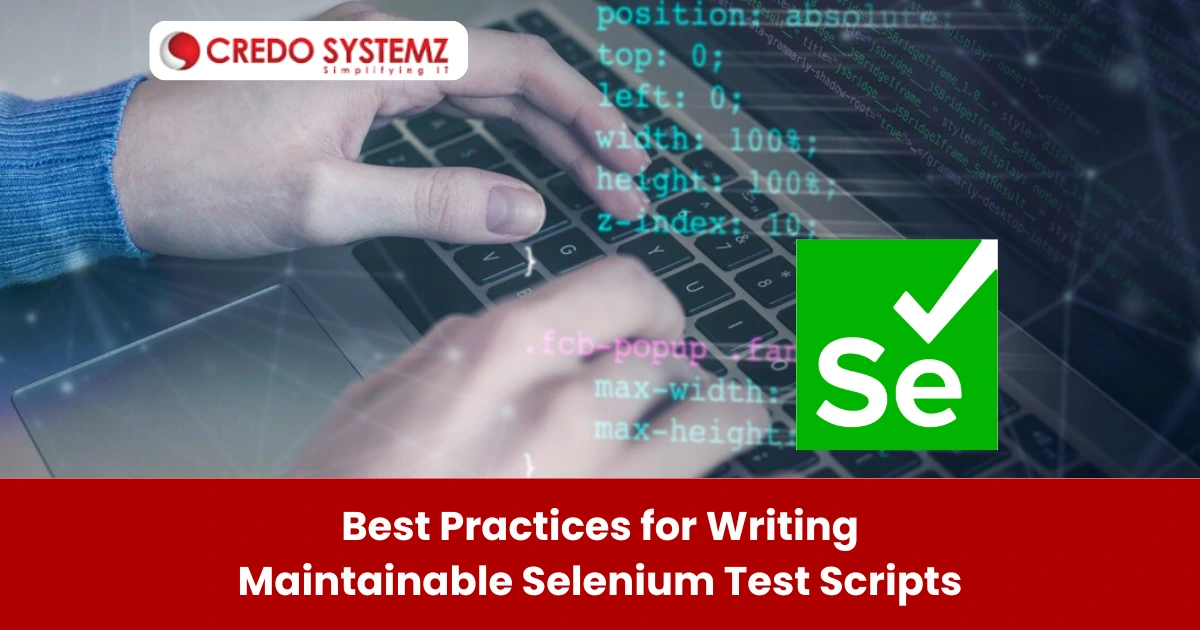
Introduction
To begin, Selenium is one of the most popular frameworks for automating web applications. Writing efficient and maintainable Selenium test scripts can be challenging. It is essential to follow best practices to ensure that the test scripts are flexible, scalable, and easy to understand. This is a detailed guide on how to write maintainable Selenium test scripts.
Follow the Page Object Model (POM) Design Pattern
The Page Object Model (POM) design pattern enhances the maintainability and readability of test scripts. The main concept of POM is to separate the logic of the test from the structure of the web page. It improves readability by abstracting away complex UI interactions. Just update the page object class when UI changes occur.
Implementation of POM
- Create a page object class for each web page or section.
- Define web elements and actions as methods in these classes
Use Explicit Waits Instead of Implicit Waits
Explicit waits are more reliable as they allow the script to wait for a specific condition before proceeding.
Benefits
- Improves stability by avoiding unnecessary delays and only waiting as long as needed.
- Reduces flakiness as Selenium waits until elements are clickable or visible.
Use Data-Driven Testing (DDT)
Data-driven testing separates test data from the test scripts that ensure easier test runs with different sets of inputs. It reduces code duplication as one script can run with multiple data sets. DDT makes tests scalable and adaptable to new data sets.
Implementation of DDT
- Store test data in external files, like Excel, CSV, JSON, or database.
- Use tools like Apache POI for Excel and Jackson for JSON parsing in Java.
Use Assertions Wisely
To verify the correctness of the test cases, Assertions are crucial but overusing or misusing them.can lead to cluttered code.
Best practices
Keep assertions simple and verify only the essential outcomes in each test. Use soft assertions that allow tests to continue running after a failure. It helps in gathering more information about a failure in large test suites.
Modularize Your Code
Break your code into smaller, reusable functions, so that one method can perform one specific action.
Advantages
Modular functions can be reused across multiple test cases that results in increased reusability. Smaller functions are easier to debug, and changes can be made in one place.
Use a Robust Test Framework
Building the Selenium tests on a robust test framework like TestNG or JUnit is a good practice. These frameworks provide:
- Annotations
- Report generation
- Test grouping
- Parallel execution capabilities
Benefits
- Improve the test organization through annotations like @BeforeMethod, @AfterMethod, and @Test.
- In-built reporting and logging mechanisms.
- Easy integration with CI/CD tools like Jenkins
Leverage Dependency Injection for WebDriver
Instead of directly instantiating the WebDriver in the tests, use dependency injection (DI). Frameworks like Spring or Guice can handle WebDriver initialization, making your code cleaner and testable.
Advantages
- Better test isolation: DI allows users to manage multiple browsers or WebDriver instances easily.
- Code reuse: Centralized management of WebDriver setup, teardown, and configuration.
Logging and Reporting
Integrating logging into the tests to improve test transparency and troubleshooting issues. To capture test execution details, use logging libraries like Log4j or SLF4J.
Best practices
Log significant steps by including details like test start, end, pass, fail, and critical actions. Combine with reporting tools like Allure, ExtentReports and TestNG’s in-built reporting for easy-to-read reports. Version Control for Test Scripts
Store the Selenium test scripts in a version control system (VCS) such as Git. This allows for:
- Collaboration among team members.
- Easy rollback to previous versions when bugs are introduced.
- Tracking changes and updates in the test scripts.
Conclusion
Finally, Writing maintainable Selenium test scripts requires coding skills, right tools, good design principles, and following best practices. To develop the skills to write selenium test scripts, join Credo Systemz Selenium training in Chennai. By implementing various important guidelines, build a robust test automation framework that scales with the application and adapts to frequent changes efficiently.
Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.