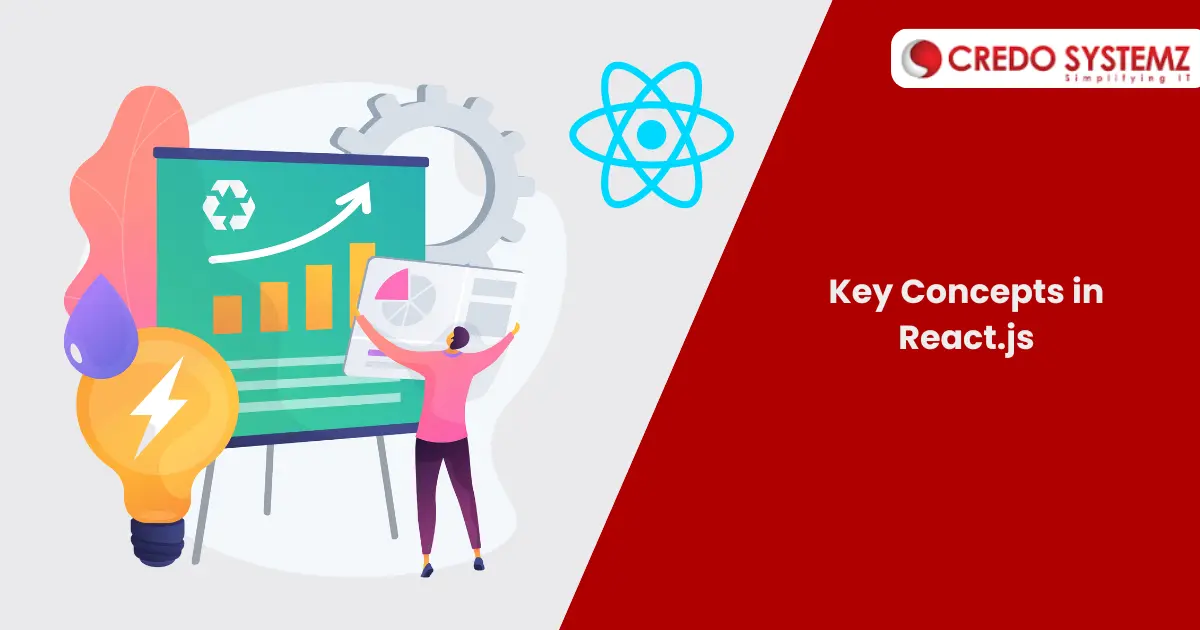
Introduction
To create interactive web applications, React.js offers a powerful and efficient way. The three fundamental concepts of React.js are: Props, State, and Lifecycle Methods. Let’s delve into these concepts to grasp their significance in React development.
Props
Props is short for properties. It is a mechanism for passing data from parent to child components. They are ready only and immutable. This is input and can be passed down the component tree.
In React, props are accessed via the this.props object within a class component. Here’s a example of how props are used:
// Parent Component class ParentComponent extends React.Component { render() { return ; } } // Child Component const ChildComponent = (props) => { return Hello, {props.name}! };State
Basically, State represents the internal state of a component. It is used for dynamic data that can change over time. Unlike props, state is mutable and controlled by the component itself. When the state of a component changes, React automatically re-renders the component and updates the UI to reflect the new state.
State is initialized in a class component using the this.state object. It can be updated using the setState() method. Here’s an example demonstrating state usage:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount = () => { this.setState({ count: this.state.count + 1 }); }; render() { return ( ); } }Count: {this.state.count}
Lifecycle Methods
Lifecycle methods are special methods that are invoked at specific points in the lifecycle of a React component. They allow developers to perform certain actions such as:
- Setting up and cleaning up resources
- Handling side effects
- Optimizing performance
It provides developers a way to manage the state and behavior of their components throughout their lifecycle. It’s essential to understand when each method is called and how they can be used to build robust and efficient React applications.
The commonly used lifecycle methods include:
- componentDidMount()
- componentDidUpdate()
- componentWillUnmount()
These methods provide hooks for executing code at different stages of a component’s life. Here’s an example illustrating the use of lifecycle methods:
class MyComponent extends React.Component { componentDidMount() { // Perform initialization tasks console.log('Component mounted'); } componentDidUpdate(prevProps, prevState) { // Perform update tasks console.log('Component updated'); } componentWillUnmount() { // Perform cleanup tasks console.log('Component will unmount'); } render() { return Hello, React!; } }The main categories of lifecycle methods in React are:
- Mounting
- Updating
- Unmounting
- Error Handling
Mounting
constructor()
This method is called when a component is initialized. It is used for initializing state and binding event handlers.render()
This method is responsible for rendering the component’s UI.componentDidMount()
This method is called after the component is mounted to the DOM. It is used for fetching data from an API.Updating
static getDerivedStateFromProps()
This is a static method that is called when the component receives new props. It is used to update the state based on the props.shouldComponentUpdate()
This method is called before rendering when new props or state are received. It allows you to control whether the component should re-render or not.render()
Same as in the mounting phase.getSnapshotBeforeUpdate()
This method is called right before the changes from the virtual DOM are to be reflected in the DOM. It allows you to capture some information from the DOM before it is potentially changed.componentDidUpdate()
This method is called after the component’s updates are flushed to the DOM. It is used for performing side effects after a component has been updated.Unmounting
componentWillUnmount() This method is called just before a component is unmounted from the DOM. It is used for cleanup tasks such as removing event listeners or canceling timers.
Error Handling
static getDerivedStateFromError() This static method is called when an error is thrown during rendering. It allows the component to render a fallback UI.
componentDidCatch()
This method is called when an error is caught during rendering. It allows you to perform side effects after an error has occurred.Conclusion
Finally, Understanding the key concepts of React—Props, State, and Lifecycle Methods—is crucial for mastering React.js development. To expertise in React.js, Credo Systemz offers the Effective React JS Training in Chennai. This React JS Course includes Professional trainers, practical training, industrial skills and placement assistance. By leveraging these features effectively, developers can build scalable, maintainable, and performant React applications.
Latest Post