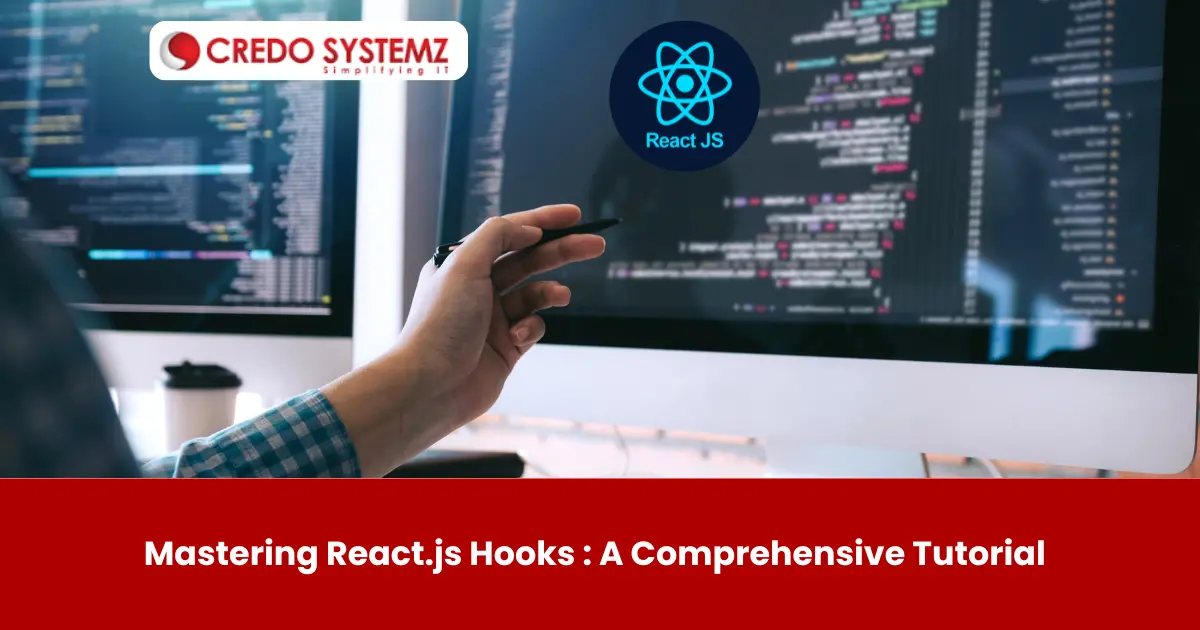
Introduction
In the web development field, React.js is the most popular JavaScript library for building user interfaces. React js hooks are a popular choice for developers to build various applications efficiently. They are introduced in React version 16.8 to use state and other React features in functional components. This article provides a comprehensive tutorial on mastering React.js Hooks with practical applications.
React Hooks
React Hooks are functions that React development experience to build efficient, modular React applications. They will “hook into” React state and life cycle features from function components.
Benefits of React Hooks
React hooks provide a modern way to build React applications with various benefits, such as:
- Allows to use state and other React features without the need for class components.
- Custom hooks extract and reuse stateful logic across multiple components.
- Achieves code readability by organizing logic based on functionality.
- Easy state management using useState and useReducer hooks.
- useEffect handles side effects like data fetching, subscriptions in a declarative way
- useMemo and useCallback optimize performance by preventing unnecessary re-renders and computations.
- Consistent way to handle stateful logic and side effects across different components.
Basic Hooks
The few commonly used React.js hooks are given below,
- useState
- useEffect
- useContext
- useReducer
- useMemo
- useRef
- useCallback
- useLayoutEffect
1. useState
To add state to functional components.
2. useEffect
Allow to perform side effects in function components. Runs code after the component renders.
3. useContext
To use context values in function components. 4. useReducer
Managing complex state logic similar to Redux. 5. useMemo
Memorizes a value that eliminates recomputing on every render.
value – recalculated only when a or b change. 6. useRef Creates a mutable object that can be used to access DOM elements. This object doesn’t cause re-renders when updated. myRef.current can hold a value without causing re-renders. 7. useCallback The function updates only when dependencies change. 8. useLayoutEffect After all DON updates, runs synchronously like ‘useeffect’.
React allows custom Hooks to encapsulate and reuse stateful logic across multiple components. To create a customer hook, define a function by starting with ‘use’. The best practices for creating a customer hook are,
To wrap up, React Hooks is a valuable skill to develop React applications by simplifying the front-end development process. To master the hooks like, useState, useEffect, useContext, useReducer, and custom Hooks, join Credo Systemz React JS Training in Chennai. This live React course ensures gaining coding skills through real-time projects and practices. Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.li>state current state
Custom Hooks
Conclusion