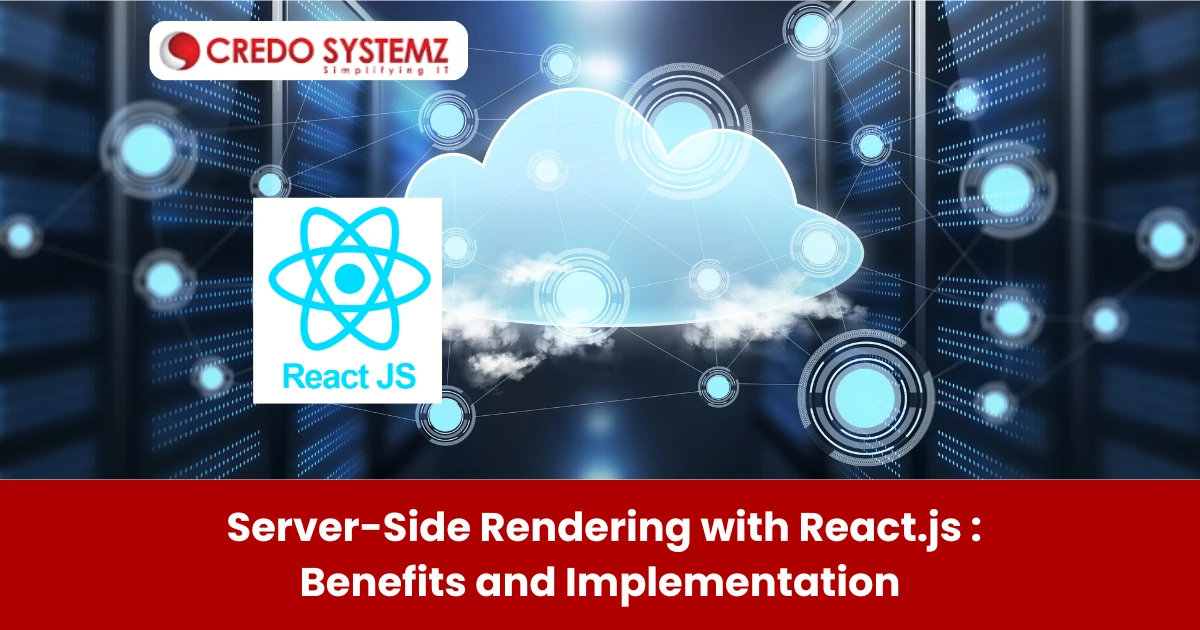
Introduction
In modern web development, server-Side Rendering (SSR) approach has gained popularity with frameworks like React.js. It is used to render React components on the server. SSR can improve performance, SEO, and initial page load time, as the HTML content is fully rendered and sent to the browser. Let’s explore the benefits of SSR with React.js through basic implementations.
Implementation of Server-Side Rendering with React.js
The basic steps to implement SSR in a React.js application are:
- Create a new React project
- Basic React Application
- Setting up the Express Server
- Client-side Hydration
- Webpack Configuration for Client-Side Bundling
- Babel Configuration
- Build the Client Bundle
- Running the Application
1.Create a new React project
First, create a basic React project by initializing it manually.Then Install the necessary dependencies:
2.Basic React Application
To create a basic React application, inside your project directory, set up the following:?[?
Create src/App.js: import React from 'react'; const App = () => { return ( ); }; export default App;Hello, Server-Side Rendering with React by Credo Systemz!
3.Setting up the Express Server
Next, set up an Express server to render the React app on the server and send the rendered HTML to the client.
Create server.js: import express from 'express'; import React from 'react'; import ReactDOMServer from 'react-dom/server'; import App from './src/App'; const app = express(); app.use(express.static('public')); app.get('*', (req, res) => { const content = ReactDOMServer.renderToString( ); const html = ` SSR React App ${content}`; res.send(html); }); app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); });
4.Client-side Hydration
After the React app is rendered on the server-side, it needs to be hydrated on the client-side to make it interactive. This can be done with React’s hydrate method.
Create src/index.js: import React from 'react'; import { hydrate } from 'react-dom'; import App from './App'; hydrate( , document.getElementById('root'));
5. Webpack Configuration for Client-Side Bundling
To bundle the client-side React code, use Webpack as it can be served from the server and loaded by the client’s browser.
Install Webpack and Babel:bash Copy code npm install webpack webpack-cli babel-loader @babel/core @babel/preset-react @babel/preset-env Create webpack.config.js: js Copy code // webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', // Client-side entry point output: { filename: 'bundle.js', // Output bundle path: path.resolve(__dirname, 'public'), // Path to serve the bundle }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { }, }, }, ], }, mode: 'development', };
6. Babel Configuration
Set up Babel to transpile the modern JavaScript and JSX syntax to code that can run in the browser.
: { "presets": [ "@babel/preset-env", "@babel/preset-react" ] }
7. Build the Client Bundle
Next, to bundle the client-side code, run Webpack by:
Adding a build script in package.json, Run the build script. "scripts": { "build": "webpack --config webpack.config.js" } npm run build
This will generate a bundle.js file inside the public directory.
8.Running the Application
Finally, run your server to start rendering the React app on the server and serving the bundle to the client. To start the server, add a start script to package.json:
“scripts”: { “start”: “node server.js”, “build”: “webpack –config webpack.config.js” } Then run: npm run build npm startVisit http://localhost:3000 in the browser. The React app will be rendered on the server and then hydrated on the client side.
Summary
When the user requests the page, Express renders the React app to HTML using ReactDOMServer.renderToString(). The client loads the HTML, and React is then hydrated on the client-side using hydrate(). Once React is hydrated, the app becomes interactive, and React takes over the rendering on the client side.
Benefits of Server-Side Rendering with React.js
The important benefits of Server-side rendering with React.js are:
- Improved SEO
- Faster Time to First Paint (TTFP) and Time to Interactive (TTI)
- Better User Experience (UX)
- Content Preloading
Improved Performance on Low-End Devices
The primary reason for adopting SSR is to enhance SEO with fully rendered HTML pages. SSR improves the initial load performance by reducing the time to first paint. Faster initial rendering enhances the overall user experience. SSR can also help with preloading content for users. The computational burden on low-powered devices is reduced by heavy rendering.
Conclusion
To conclude, Server-side rendering with React.js offers improved performance, SEO, and UX benefits. By following the basic implementation steps, take advantage of SSR to enhance your React applications. To gain the skills of React.js, join Credo Systemz React JS Training in Chennai
Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.