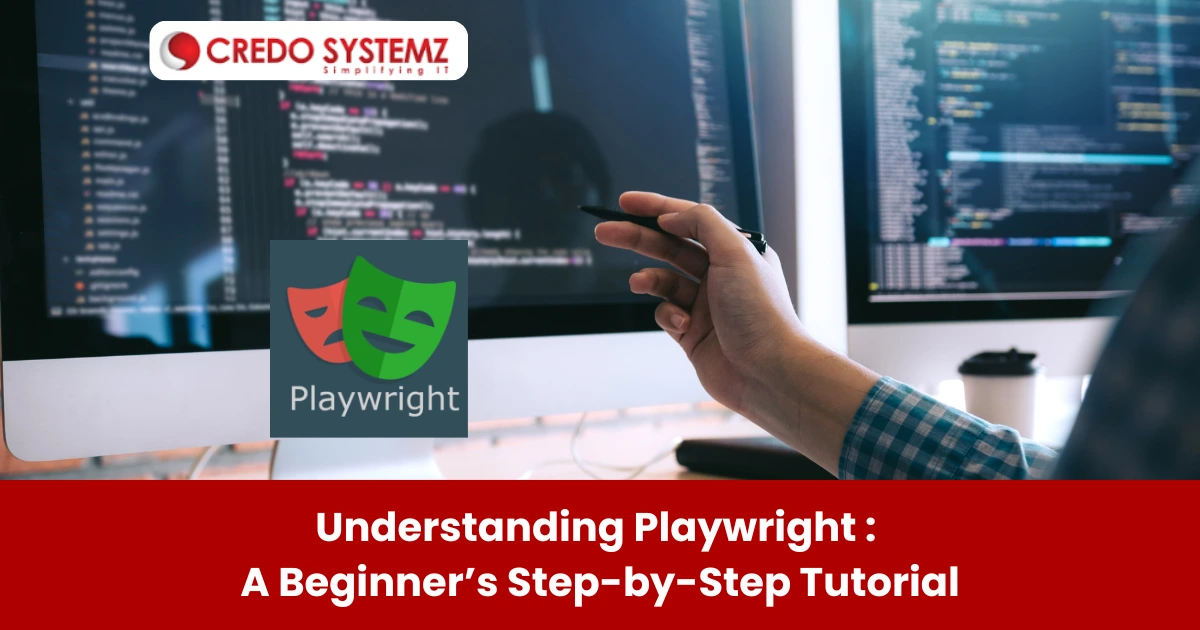
Introduction
In the modern web development field, testing applications is crucial across multiple browsers. Playwright is an open-source Microsoft automation library which simplifies end-to-end testing. This article guides you to understand playwright through the fundamentals of Playwright.
Playwright
Playwright is a Node.js library designed for automated testing of web applications. It supports multiple browsers, including Chromium, Firefox, and WebKit. Developers can write reliable tests using Playwright that runs in real browsers. It enables better cross-browser compatibility testing.
Key Features of Playwright:
The important features of Playwright are:
- Multi-browser support
- Headless and headful modes
- Auto-wait feature
- Parallel test execution
- Network interception.
Playwright works across various browsers, like Chromium, Firefox, and WebKit. It can run tests in both UI and non-UI modes. Playwright waits for elements to be available before interacting. It runs multiple tests simultaneously to reduce test execution time. Playwright allows mocking and modifying network requests for better testing.
Installation of Playwright
To use Playwright, install Node.js by downloading it from Node.js official website.
After installing, open the terminal or command prompt and run the command:
Alternatively, install Playwright along with browsers using:
This command installs Playwright with the necessary browser binaries.
Creating a Basic Playwright Test
The important steps involved in creating a simple test script is to launch a browser and navigate to a webpage.
Create a new JavaScript file and add the following code:
const { chromium } = require('playwright'); (async () => { // Launch the browser const browser = await chromium.launch({ headless: false }); // Open a new page const page = await browser.newPage(); // Navigate to a webpage await page.goto('https://example.com'); // Take a screenshot await page.screenshot({ path: 'screenshot.png' }); // Close the browser await browser.close(); })();
Run the script using:
node test.js
This script will launch a Chromium browser and navigate to example.com. Take a screenshot and close the browser.
Writing an Automated Test
Playwright has its own test runner, Playwright Test. It can be used with testing frameworks like Jest, Mocha.
1.Installing Playwright Test
To install Playwright Test:
2.Creating a Test File
Create a new test file, example.test.js, and add:
const { test, expect } = require('@playwright/test'); test('Basic test example', async ({ page }) => { await page.goto('https://example.com'); // Check if the title contains "Example Domain" const title = await page.title(); expect(title).toContain('Example Domain'); });
3.Running the Test
Run the test using:
This command will execute the test and display results in the console.
Running Tests in Different Browsers
Playwright allows running tests in multiple browsers. Modify playwright.config.js and add:
module.exports = { projects: [ { name: 'Chromium', use: { browserName: 'chromium' } }, { name: 'Firefox', use: { browserName: 'firefox' } }, { name: 'WebKit', use: { browserName: 'webkit' } }, ], };
Now, run tests in all browsers using:
- npx playwright test –project=all
- Debugging and Reporting
Run Playwright in debug mode using:
npx playwright test --debug
This opens the browser in interactive mode and allows us to inspect elements.
Playwright supports HTML reports using the command:
npx playwright test --reporter=html
View the report by opening playwright-report/index.html in a browser.
Conclusion
Finally, Playwright is a powerful tool for automating web testing across different browsers. We have covered the basics from installation, writing simple tests to running tests in different browsers, and debugging. To learn Playwright with its advanced features like API testing, mobile emulation, and integration with CI/CD pipelines, Credo Systemz provides the Playwright training course in Chennai. Start experimenting with Playwright and life up your testing career!
Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.