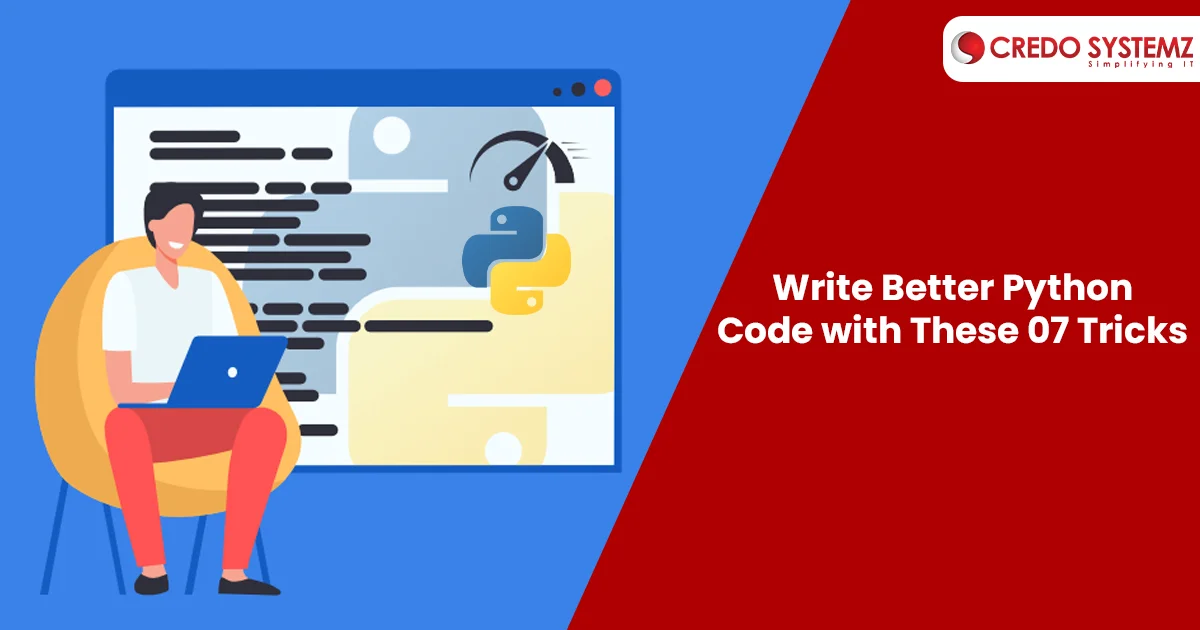
Introduction
Python is one of the popular programming languages for beginners to learn with a rise in interest by 27% from the previous year according to O’Reillyreport. Learning python coding is fun because the same functionalities can be achieved in many different ways. Anyone can learn Python and become a Python Developer with practice.
Indexing
In python, we have compound datatypes to store multiple items of different type of data, such as,
- List
- Tuple
- Set
- Dictionary
To access individual items in compound data types, we can use indexing using a numerical index in square brackets. Like other main stream programming languages, Python supports 0-based indexing, where we access the first element using zero within a pair of square brackets.
Program
# Positive Indexing
alphabet = [a,b,c,d,e,f,g]
print("First Alphabet:", alphabet[0])
print("First Four Alphabet :", alphabet [:4])
print("Odd Alphabet:", alphabet [::2])
Output
First Alphabet: a
First Four Numbers: [a, b, c, d]
Odd Numbers: [a, c, e, g]
Python also support negative indexing in which -1 refers to the last element in the sequence and using that we can count the items backward. For example, the last but one element has an index of -2 and so on. Importantly, the negative indexing can also work with the positive index in the slice object.
Program
# Negative Indexing
values= (10, 40, 4)
names = ["Priya", "Anu", "Rajkumar", "Danny"]
print(values[-1])
print(names[-3:-1])
Output
4
[‘Anu’, ‘Rajkumar’]List of Strings creation
Strings are identifiers for storing sequence of characters and individual characters of a String can be accessed by using the method of Indexing. To create list of strings use Compound datatypes like list
split() method
The split () method breaks the given string into multiple strings at the specified separator arranges them in a list and returns the list. Program
text = 'Python for all'
print(text.split(' '))
Output
[‘Python’, ‘for’, ‘all’]
As shown above,by default the split() method uses the spaces as the separators and creates a list of strings from the given string. We can use a different kind of separator like commas to create a list of strings with some elements containing spaces.
Program
txt = ‘hello, how r u, glad to meet you’
x = txt.split(", ",4)
print(x)
Output
[‘hello’,’how r u’,’glad to meet you]
“, “- separator
4- maxsplit,/p> The separator,maxsplit are the two parameters used in the split() method to specify where the splits occur and the maximum number of splits. These parameters are optional. If the parameter values are not provided, the string splits at whitespaces and no limit on the number of splits.
Ternary Expression
To check condition or todefine variables with particular condition, we use control flow statements like if…else statement
Program
if mark >90
reward=’excellent’
else:
reward=’good’
We can use ternary expression to check the condition while dealing with assignment of one variable with few lines of code.
The syntax for the ternary operator in Python is:
[on_true] if [expression] else [on_false]
Let’s rewrite the above code using ternary operator,
reward=’excellent’ if mark>90 else reward=’good’Learn Python and Certified – Get Your Certification
With Statement for File Object
We can read data from files and write data to files. To open a file use the built-in open() function, which creates a file object that can operate. Let’s see the below scenario,
Create a text file that has the text: Hello World!
Close the file
Open the file and append new data
Close the file
If we don’t close the file after any changes like writing new data, editing new data, the changes won’t be saved. We can do this using the “with” statement, which will close the file for us automatically. Program
with open("hello_world.txt", "a") as file:
file.write("Hello Python!")
with open("hello_world.txt") as file:
print(file.read())
print("Is file close?", file.closed)
Hello World!HelloPython!Hello Python!
Is file close? True
Using the with statement,the file gets closed automatically.Evaluate Multiple Conditions
There are several possible scenariosto evaluate multiple conditions. We can have multiple comparisons for the same variable for numeric values.
if x < 5 and x> 1:
pass
if 1 < x < 5:
pass
Use the in keyword to have multiple equality comparisons:
if a == "Jan" or a == "Feb" or a == "Mar" :
pass
if b in "Jan Feb Mar".split():
pass
Use the built-in functionsall() and any() for evaluating multiple conditions. The all() function will evaluate to be True when the elements are all True, and suitable to replace a series of AND logical comparisons. On the other hand, the any() function will evaluate to be True when any element is True, and suitable to replace a series of OR logical operations.
Use Default Values in Function Declarations
Most of the python projects involve creating and calling functions multiple times whenever needed. Functions are used to implement program logic to execute repeatedly at different places in the code. Arguments are used to pass data to these functions and default argument values can be used in Python functions. Parameters are the values passed to the function arguments and operate depending on the parameters.
Syntax
deffunction_name(param1=value1, param2, param3)Sorting With Different Order Requirements
Sorting is essential utility for any programming language. The basic sorting is based on the numeric order, and can use the built-in sorted() function. The sorted() function will sort the list in the ascending order. If we specify the reverse argument to be True, we can get the items in the descending order.
Program
# A list of numbers and strings
numbers = [1, 3, 5, 2, 6, 4]
words = ['orange', 'apple', 'papaya', 'mango']
# Sort them
print(sorted(numbers))
print(sorted(words))
Output
[1, 2, 3, 4, 5, 6]
['apple', 'mango', 'orange', 'papaya']
Program
# Sort them in descending order
print(sorted(numbers, reverse=True))
print(sorted(words, reverse=True))
Output:
[6, 5, 4, 3, 2, 1]
[ 'papaya', 'orange', 'mango', 'apple']
Conclusion
To conclude, this article clearly explains the few important tricks to Write Better Python Code with easy explanation. To know more and learn with the help of professional expert trainers: Python Certification course with Placements