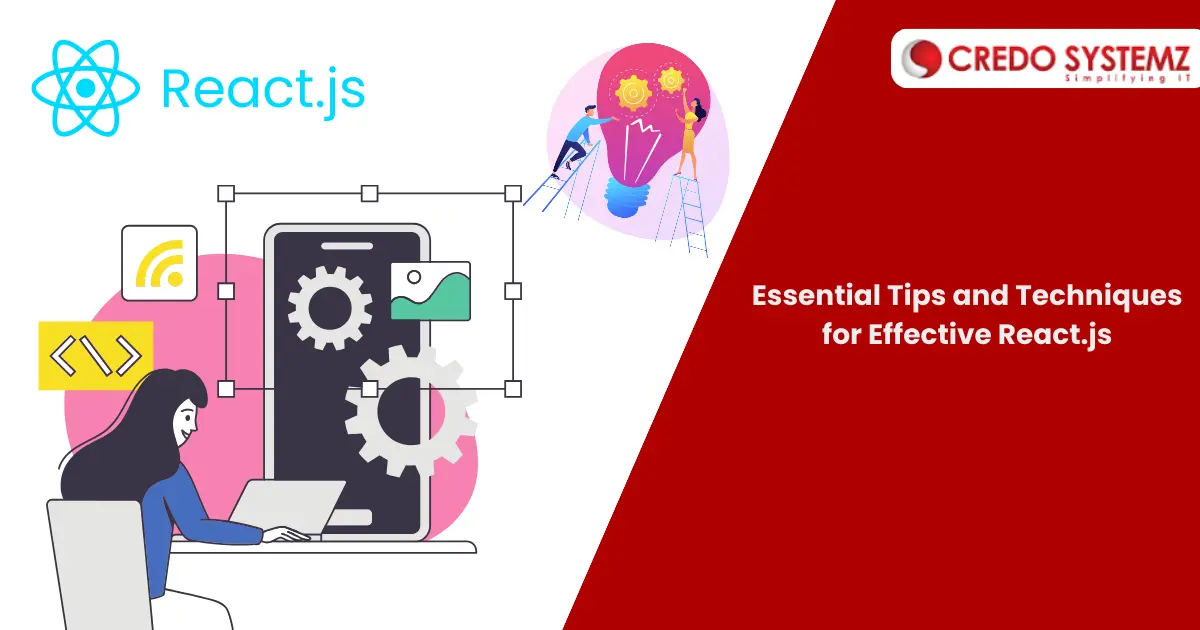
Introduction
In the web development field, React.js stands out as the most popular JavaScript libraries for building user interfaces. It allows us to build reusable UI components. To build efficient and maintainable React code, developers need to follow best practices. This article delves into essential tips and techniques for effective React.js development, such as:
Component Structure and Design Patterns
- Component Structure and Design Patterns
- State Management
- React Hooks
- Performance Optimization
- Redux Toolkit
- User Experience
Component Structure and Design Patterns
Understanding how to structure components and utilize design patterns can significantly enhance the development workflow.
- Organize the components into small, reusable pieces. Each component should have a single responsibility.
- Separate the logic and UI by using container components and presentational components.
- Adopt a consistent file structure. Follow a clear and consistent naming convention for the files and components.
State Management
- Effective state management is crucial for building robust and maintainable React applications.
- Use local state for managing state within a single component like useState hook.
- Lift the state up to the nearest common ancestor and pass it down via props.
- Use React’s Context API for handling global state. It provides a way to share values between components.
- Use state management libraries like Redux or MobX for complex applications .
React Hooks
- React Hooks provide a powerful way to manage state and side effects in functional components.
- Use useState hook to add state to functional components. It keeps the components simple and clean.
- Use useEffect for side effects like data fetching, subscriptions, and manually changing the DOM.
- Extract reusable logic into custom hooks which promotes code reusability.
- Use useReducer is suitable for dealing with complex state logic.
Performance Optimization
- Optimizing performance in React applications is vital for delivering a smooth and responsive user experience.
- Use React.memo to prevent unnecessary re-renders of functional components.
- Implement code splitting and lazy loading using React.lazy and Suspense.
- Key your list items correctly to help in identifying which items have changed, added, or removed.
- Use React’s built-in Profiler to identify performance bottlenecks.
Redux Toolkit
Redux Toolkit simplifies and streamline state management in React applications. It is the recommended way to write Redux logic.
- Create slices using createSlice to generate actions and reducers automatically.
- Leverage middleware like redux-thunk for asynchronous logic.
- Use Redux DevTools for real-time state monitoring and debugging.
- Follow web accessibility standards to ensure the accessibility of applications
- Utilize CSS frameworks or libraries like Styled Components to achieve responsiveness
- Implement comprehensive error handling in the React components to catch and manage errors gracefully.
- React Transition Group and Framer Motion libraries can be used for adding animations.
Conclusion
By following these best practices, React developers can build efficient, maintainable, and high-performing applications. Each of the above aspect plays an important role in the development process. Embrace these techniques to enhance your React.js development workflow and deliver superior user experiences. To master these React.js technical skills, Credo Systemz provides the React JS Course in Chennai. Learn the latest best practices of React.js using expert trainers.
Join Credo Systemz Software Courses in Chennai at Credo Systemz OMR, Credo Systemz Velachery to kick-start or uplift your career path.